jQuery 'onImagesLoad' Plugin
Example 4 - Combination of Examples 1, 2, and 3
Code
<script type="text/javascript" src="https://code.jquery.com/jquery-latest.js"></script>
<script type="text/javascript" src="jquery.onImagesLoad.js"></script>
<script type="text/javascript">
$(function(){
var eventNumber = 1; //so we can track the ordering of the events in the example
function displayTxt(domObject){ //helper function for displaying results
return '<' + domObject.tagName + (domObject.id ? ' id="' + domObject.id + '"' : '') + '>';
}
///////////////////////// EXAMPLE 3 /////////////////////////
//attach directly to each image within each .imageSection
$('.imageSection img').onImagesLoad({
each : eachImgLoaded_example3,
all : allImgsLoaded_example3
});
//eachImgLoaded_example3 - the 'each' callback is invoked once for each individual image that loads
//i.e. the 'each' callback will be invoked "$('.imageSection img').length" times
function eachImgLoaded_example3(domObject){
//note: this == domObject. domObject will be the image element that has just finished loaded
$(domObject).parent().prepend('<div class="loaded">Event #'+(eventNumber++)+': Image ' + displayTxt(domObject) + ' has loaded. '
'Displayed dimensions: ' + domObject.width + 'x' + domObject.height + '</div>');
}
//allImgsLoaded_example3 - the 'all' callback is invoked only once: when all images that
//$('.imageSection img') encapsulates have loaded
function allImgsLoaded_example3($selector){
//note: this == $selector. $selector is $('.imageSection img') here
var allLoaded = ""; //build a string of all items within the selector
$selector.each(function(){
allLoaded = displayTxt(this) + ', ';
})
$('body').prepend('<div class="loaded">Event #'+(eventNumber++)+': The following images have loaded within selector $("'
+this.selector+'"), which contains the following ' + $selector.length + ' items: ' + allLoaded + '</div>');
}
///////////////////////// EXAMPLE 2 /////////////////////////
//attach onImagesLoad to specific divs
$('.imageSection').onImagesLoad({
each : eachItemLoaded_example2,
all : allImgsLoaded_example2
});
//eachItemLoaded_example2 - the 'each' callback is invoked once for each item that $('.imageSection') encapsulates
//i.e. $('.imageSection').length == 2 here, so the 'each' callback will be invoked twice
function eachItemLoaded_example2(domObject){
//note: this == domObject. domObject will be the <div class="imageSection" /> that has just finished loading all of its images
$(domObject).prepend('<div class="loaded">Event #'+(eventNumber++)+': All images have loaded within item '
+ displayTxt(domObject) + '</div>');
}
//allImgsLoaded_example2 - the 'all' callback is invoked only once: when all images that
//$('.imageSection') encapsulates have loaded
function allImgsLoaded_example2($selector){
//note: this == $selector. $selector is $('.imageSection') here and $selector.length == 2 (since we have 2 imageSection classes on the page)
var allLoaded = ""; //build a string of all items within the selector
$selector.each(function(){
allLoaded = displayTxt(this) + ', ';
})
$('body').prepend('<div class="loaded">Event #'+(eventNumber++)+': All images have loaded within selector $("'
+ $selector.selector + '"), which contains the following ' + $selector.length + ' items: ' + allLoaded + '</div>');
}
///////////////////////// EXAMPLE 1 /////////////////////////
//attach onImagesLoad to the entire body - invoked once when all images contained within $('body') have loaded
$('body').onImagesLoad( function($selector){
//note: this == $selector. $selector will be $("body") in this example
$selector.prepend('<div class="loaded">Event #'+(eventNumber++)+': All images have loaded within selector $("'
+ this.selector + '")</div>');
});
});
</script>
Images
<div id="imageSectionOne" class="imageSection">
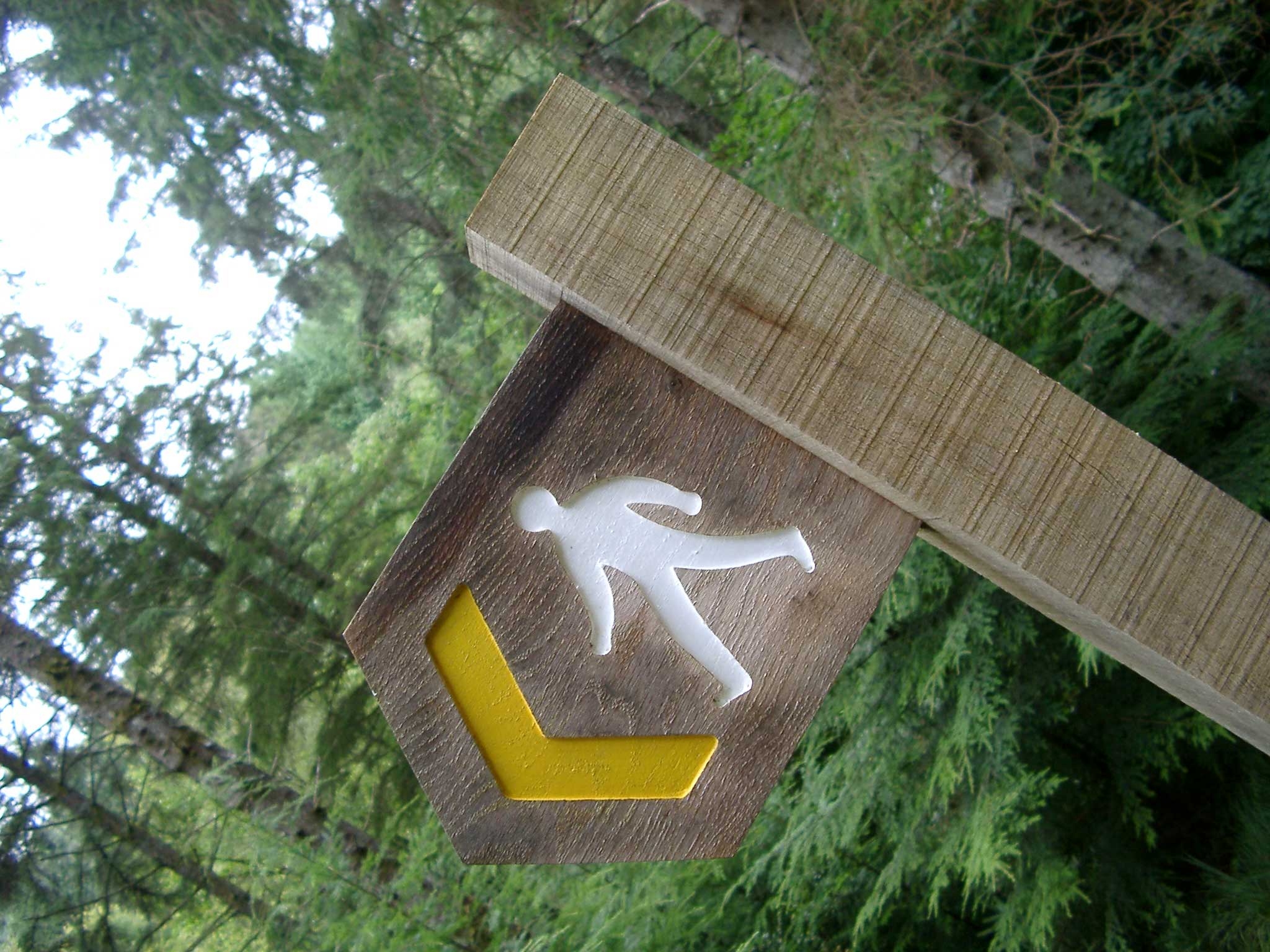
<div id="imageSectionTwo" class="imageSection">
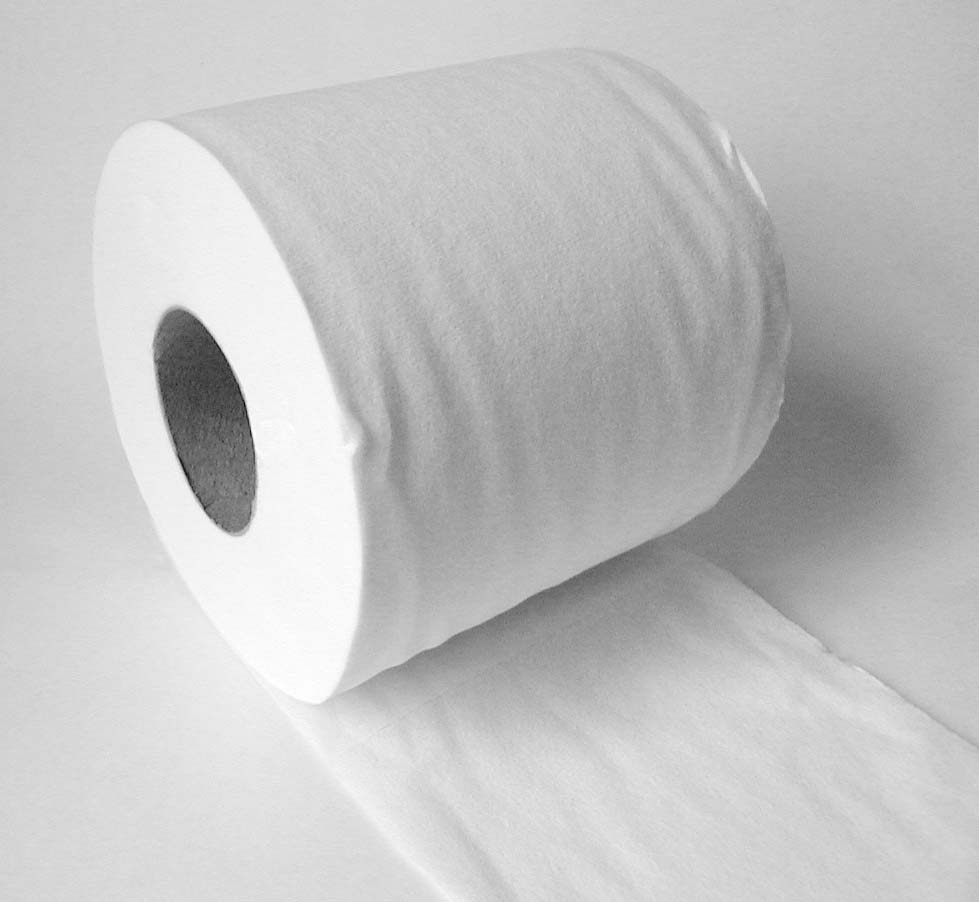
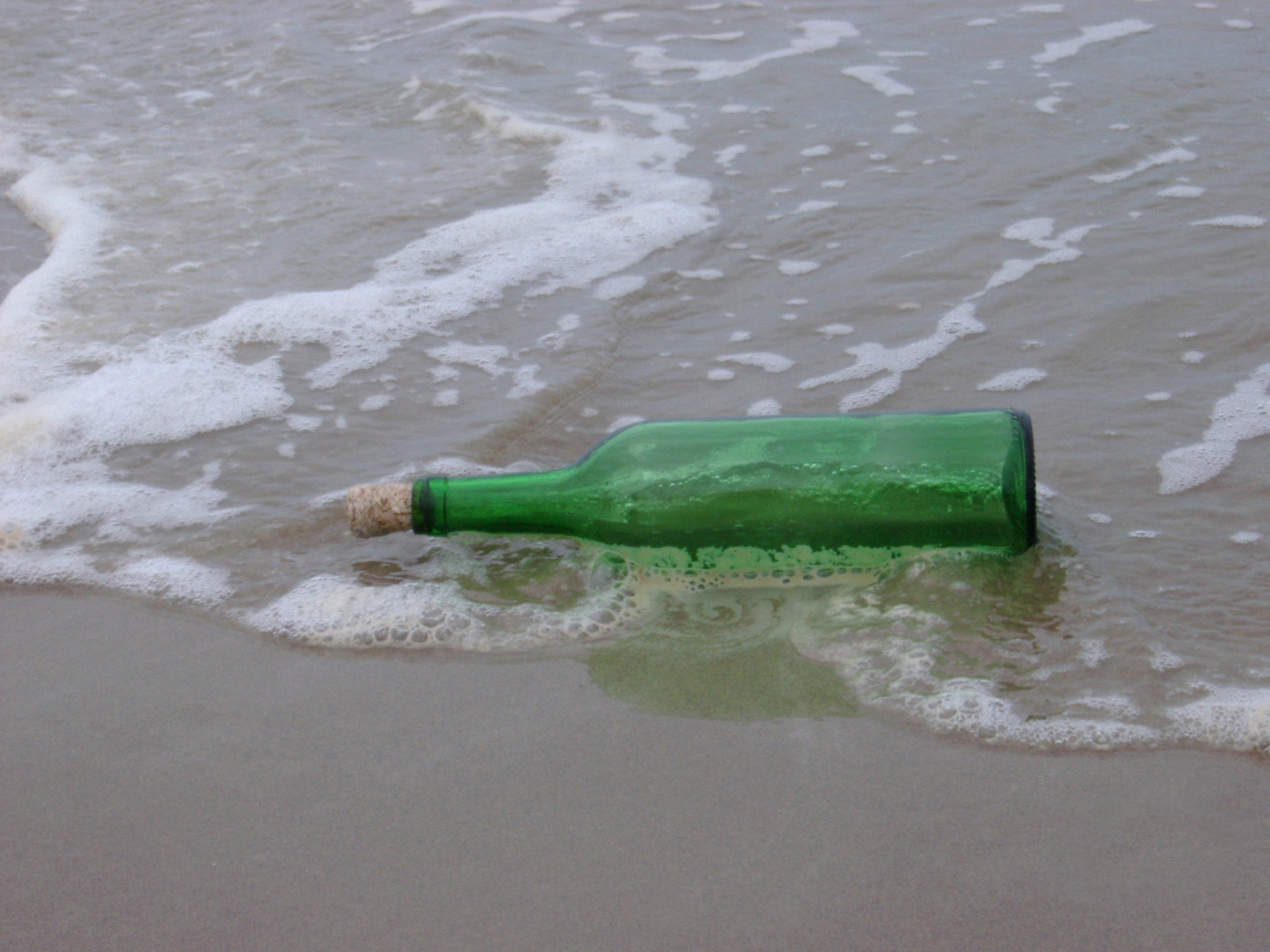
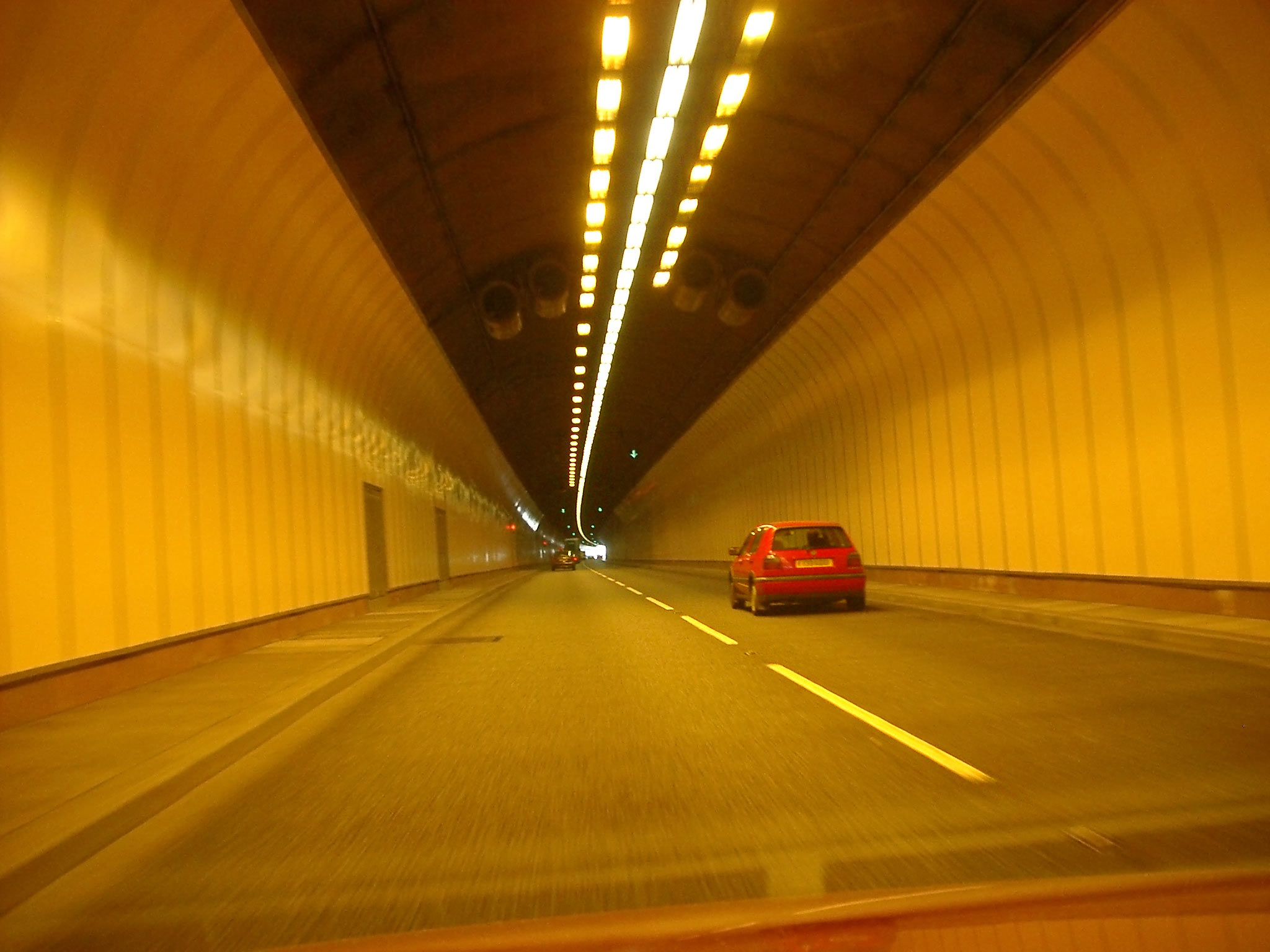