jQuery 'onImagesLoad' Plugin
Example 2 - Using the 'all' callback vs. the 'each' callback
-
The 'all' callback - invoked only once per selector: when all images within the entire selector have loaded.
-
The 'each' callback - invoked individually by each item that your selector encapsulates (can be a DIV, SPAN, IMG, etc.).
In this example, our selector is $('.imageSection'). Since there are two elements on this page containing the class "imageSection", our selector
will encapsulate those two items.
- The 'all' callback will only be invoked when ALL images within the two "imageSections" have loaded.
- In this example, the 'all' callback will prepend red text in a yellow box at the top of the body when all images within the selector "$('.imageSection')" have loaded.
- The 'each' callback will be invoked twice, once for each element on the page containing the class "imageSection".
- In this example, the 'each' callback will prepend red text in a yellow box at the top of each DIV element containing the class "imageSection" when its own images have loaded.
- Reload the page (and all images) a few times and notice how each imageSection declares it has finished loading independently of the other section.
Code
<script type="text/javascript" src="https://code.jquery.com/jquery-latest.js"></script>
<script type="text/javascript" src="jquery.onImagesLoad.js"></script>
<script type="text/javascript">
var eventNumber = 1; //so we can track the ordering of the events in the example
$(function(){
$('.imageSection').onImagesLoad({
each : eachItemLoaded,
all : allImgsLoaded
});
//the 'each' callback is invoked once for each item that $('.imageSection') encapsulates
//i.e. $('.imageSection').length == 2 here, so the 'each' callback will be invoked twice
function eachItemLoaded(domObject){
//note: this == domObject. domObject will be the <div class="imageSection" /> that has just finished loading all of its images
$(domObject).prepend('<div class="loaded">Event #'+(eventNumber++)+': All images have loaded within item ' + displayTxt(domObject) + '</div>');
}
//the 'all' callback is invoked only once: when all images that $('.imageSection') encapsulates have loaded
function allImgsLoaded($selector){
//note: this == $selector. $selector is $('.imageSection') here and $selector.length == 2 (since we have 2 imageSection classes on the page)
var allLoaded = ""; //build a string of all items within the selector
$selector.each(function(){
allLoaded = displayTxt(this) + ', ';
})
$('body').prepend('<div class="loaded">Event #'+(eventNumber++)+': All images have loaded within selector $("' + $selector.selector + '"), which contains the following ' + $selector.length + ' items: ' + allLoaded + '</div>');
}
function displayTxt(domObject){ //helper function for displaying results
return '<' + domObject.tagName + (domObject.id ? ' id="' + domObject.id + '"' : '') + '>';
}
});
</script>
Images
<div id="imageSectionOne" class="imageSection">
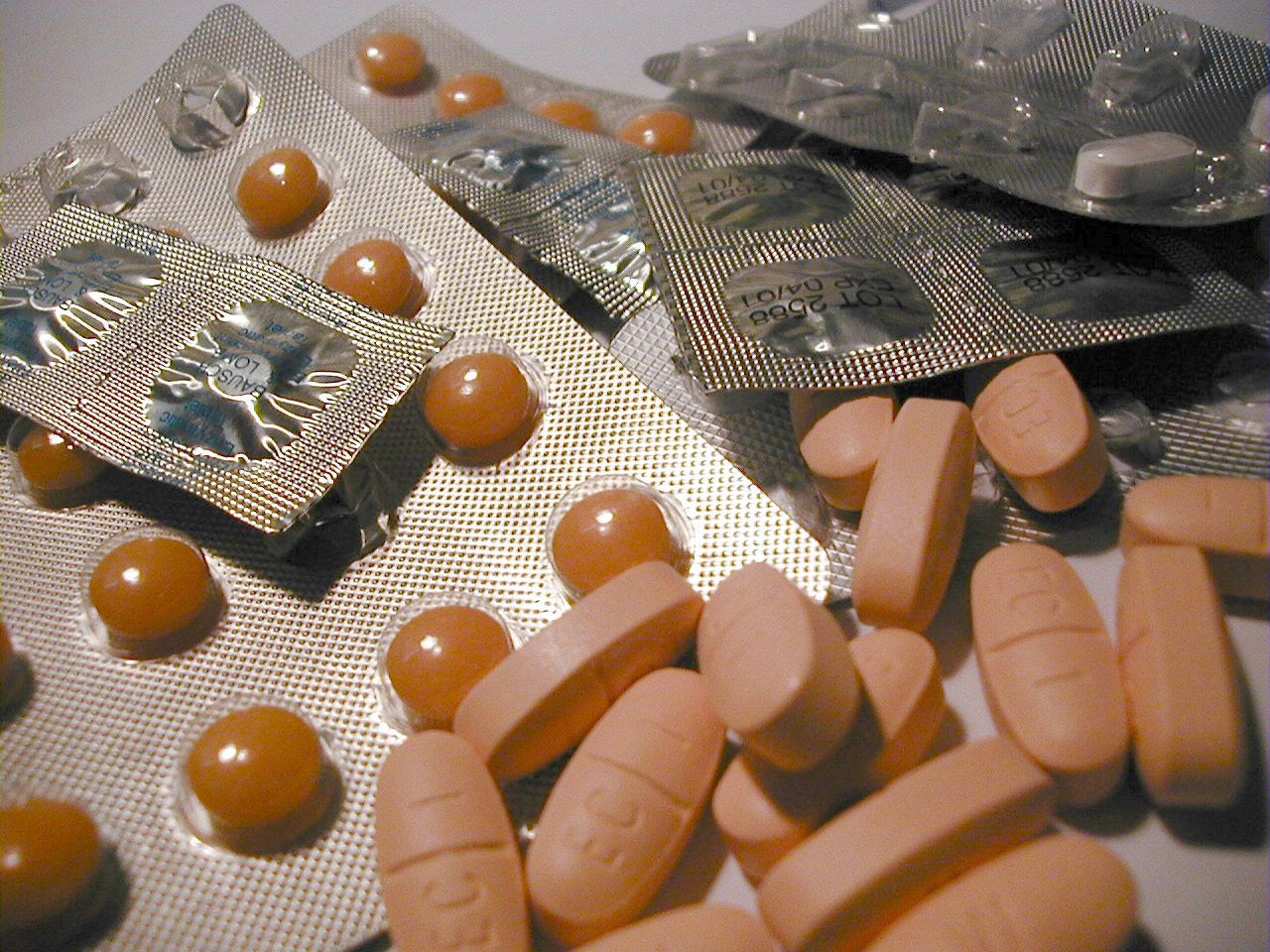
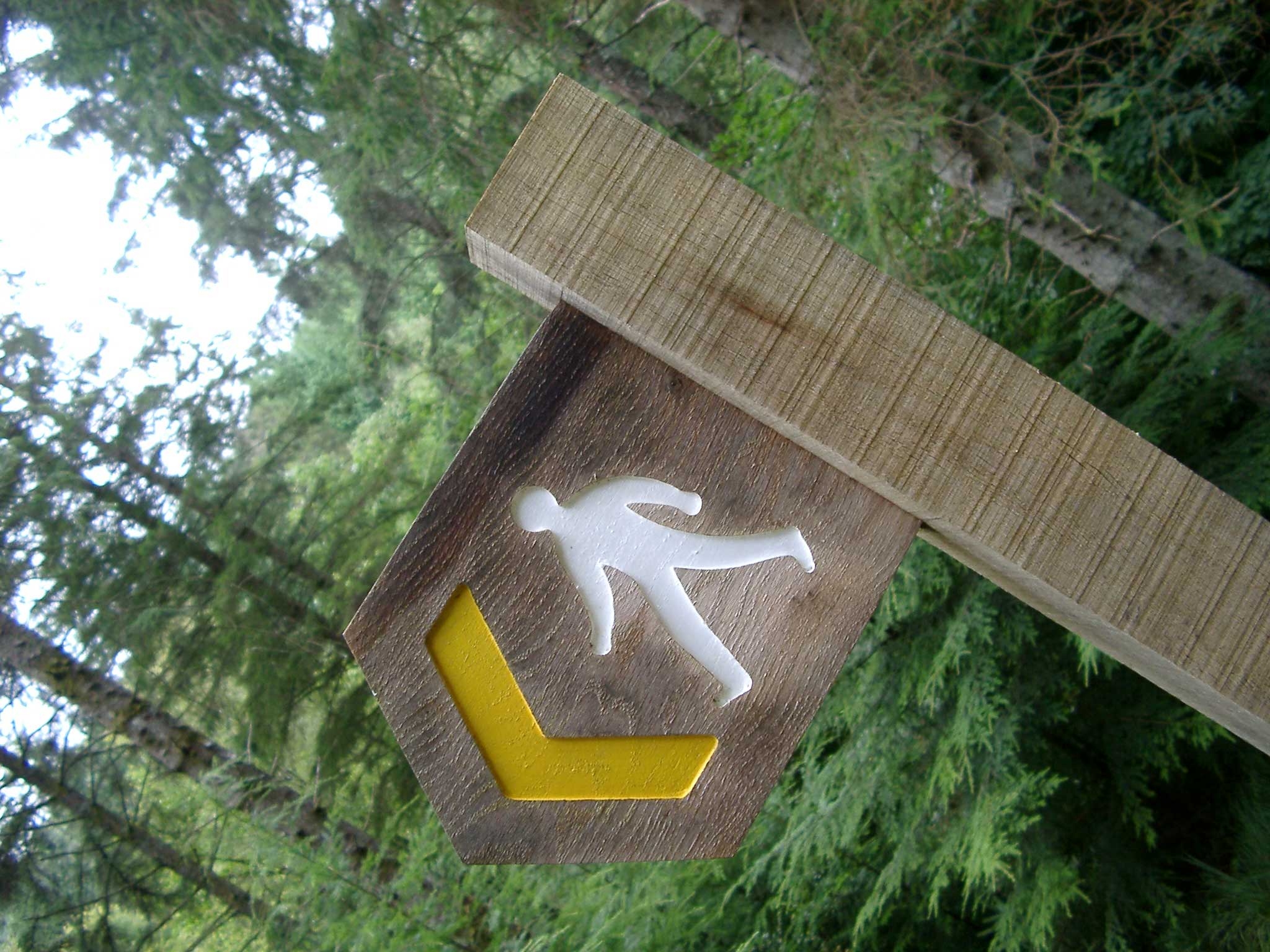
<div id="imageSectionTwo" class="imageSection">
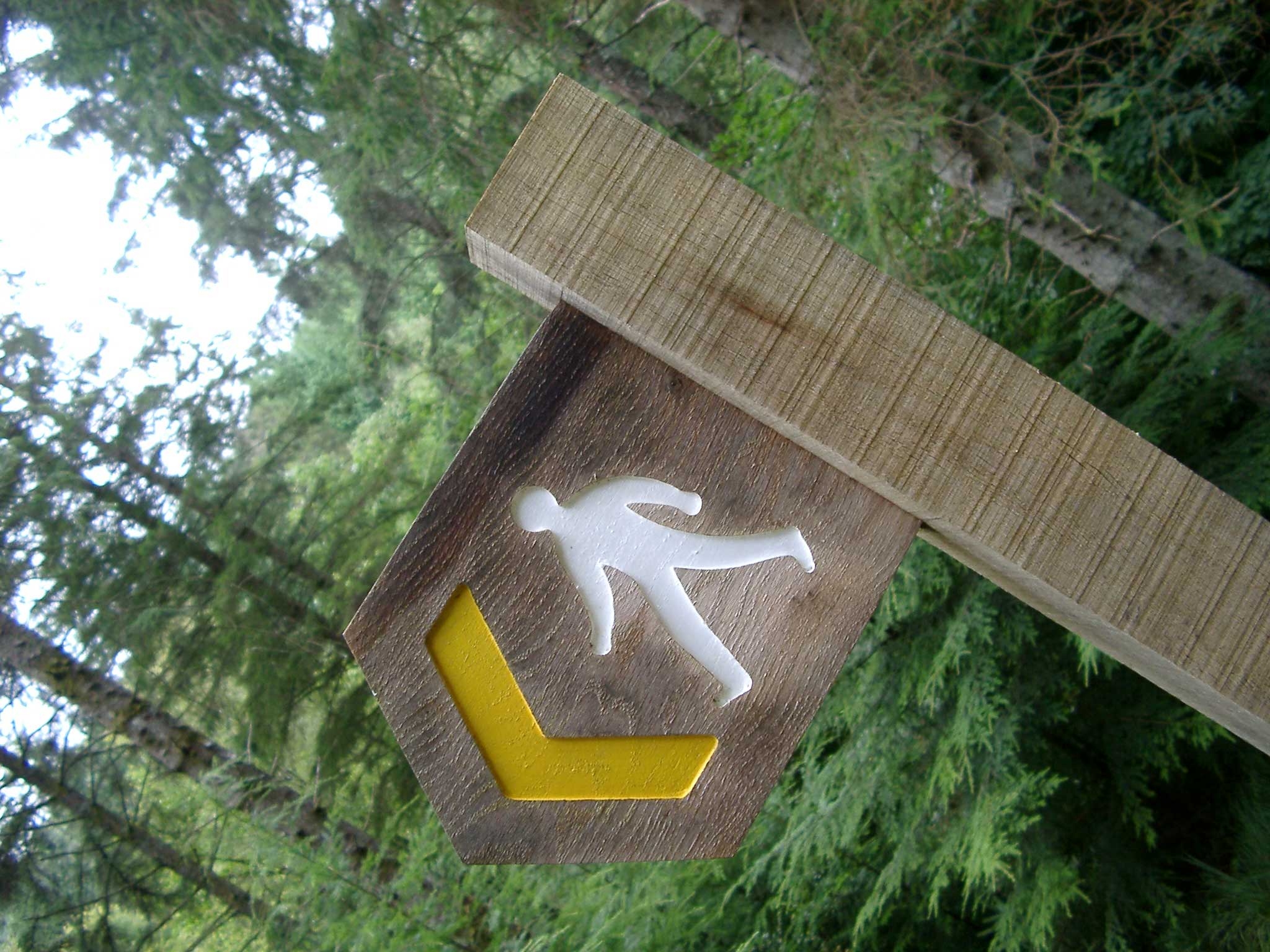